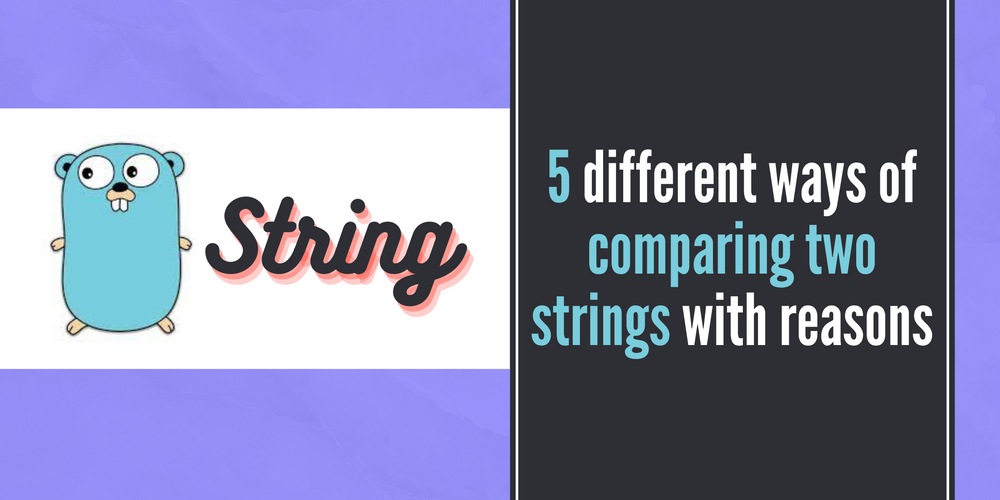
Golang - 5 different ways of comparing two strings with reasons
Learn with reason on which method to choose when you are doing the string comparison in Golang and never go wrong.-
Sriram Thiagarajan
- November 12, 2022
Golang - 5 different ways of comparing two strings with reasons
Program logic often depends on comparing strings to make a decision. In Go, strings are actually a sequence of bytes, and comparing them involves comparing the individual bytes of the string.
What method to choose
Method | Reason |
---|---|
==, != | A simple comparison between small strings |
strings.Compare() | The recommended way for comparing case-sensitive strings |
strings.EqualFold() | The recommended way for comparing case-insensitive strings |
strings.Contains() | Comparing case-sensitive substring |
strings.Contains() with lowercase | Comparing case-insensitive substrings |
How to use == for strings comparison in Golang?
You can check if the string is equal to one another using the == operator.
package main
import (
"fmt"
)
func main() {
// Check if the search string and name are same
name := "Learning Go"
searchText := "Learning Go"
if name == searchText {
fmt.Println("Same strings")
}
}
You can also use the !=
to check for inequality in golang.
package main
import (
"fmt"
)
func main() {
// Inequality check
name := "Learning Go"
searchText := "Test"
if name != searchText {
fmt.Println("Different strings")
}
}
How to compare using strings package in golang?
There is compare method in the strings package which can be used to get an integer value ranging in the following values.
- Compare() returns 0 - string are same
- Compare() returns 1 - first string is bigger
- Compare() returns -1 - first string is lesser
Method definition
func Compare(a, b string) int
Official doc for Contains() method
Example
package main
import (
"fmt"
"strings"
)
func main() {
// Check if the search string and name are same
name := "Learning Go"
searchText := "Learning Go"
if strings.Compare(name, searchText) == 0 {
fmt.Println("Same strings")
}
// Inequality check
name = "Learning Go"
searchText = "Test"
if strings.Compare(name, searchText) != 0 {
fmt.Println("Different strings")
}
}
How to compare case-insensitive strings in golang?
The EqualFold
method in the strings package can be used for case-insensitive string comparison. This method returns true if the strings are equal otherwise it will return false.
Method definition
func EqualFold(s, t string) bool
Offical docs for EqualFold() method
Example
package main
import (
"fmt"
"strings"
)
func main() {
// Check if the search string and name are same
name := "LeaRNIng GO"
searchText := "Learning Go"
if strings.EqualFold(name, searchText) {
fmt.Println("Same strings")
}
}
How to compare substrings in golang?
When you are implementing the search and filtering logic in your go program, you would need to compare the search text with a partial search in your database and return the results of the operation.
You can use the Contains()
method in the strings package to do this partial search. It returns true if the substring is present in the other string and false if not.
Method definition
func Contains(s, substr string) bool
Official docs for Contain() method
Example
package main
import (
"fmt"
"strings"
)
func main() {
// Check if the search string is present in name
name := "Learning Go"
searchText := "Learn"
if strings.Contains(name, searchText) {
fmt.Println("It contains the substring")
}
}
How to compare case-insensitive substring in golang?
You can use the contains method along with converting all the strings to lowercase to get the case-insensitive substring search in golang
Method definition
func ToLower(s string) string
Official docs for ToLower() method
Example
package main
import (
"fmt"
"strings"
)
func main() {
// Check if the search string is present in name
name := strings.ToLower("Learning Go")
searchText := strings.ToLower("learn")
if strings.Contains(name, searchText) {
fmt.Println("It contains the substring")
}
}