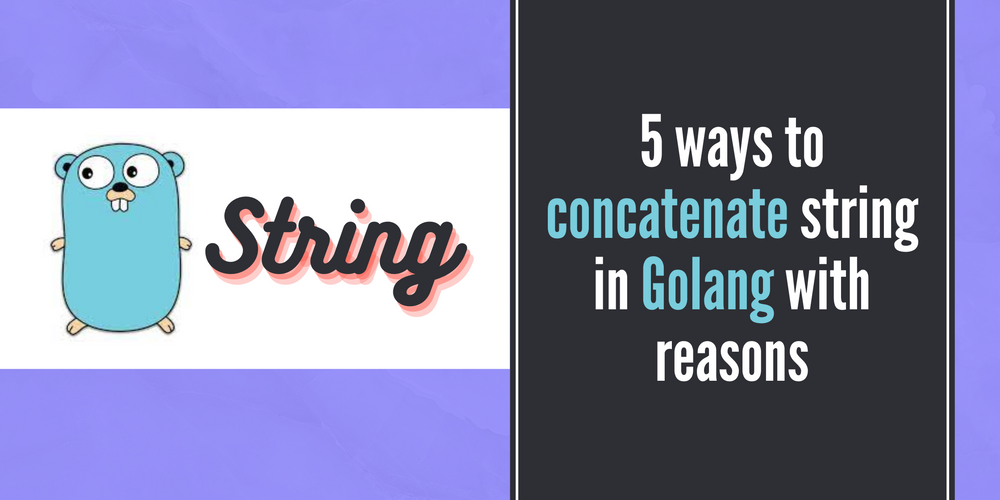
5 ways to concatenate string in Golang with reasons
Learn with reason on which method to choose when you are doing the string concatenation in Golang and never go wrong.-
Sriram Thiagarajan
- October 28, 2022
5 ways to concatenate string in Golang with reasons
String manipulation has always been a common task when you write code. You will understand the simple and performant methods to do string concatenation in this post. In Go, string is a read-only slice of bytes and so you need to use the best method to perform the concatenation based on the situation.
Reasons to choose a method
I am going to cover the primary reason for the different methods. You can choose which method to use based on your situation.
Methods | Reason |
---|---|
Simple join using + | Simple concatenation of one or two small strings |
strings.Builder | Efficient concatenation of large strings / inside loops |
bytes.Buffer | Efficient concatenation of large strings / inside loops / reading from files |
fmt.Sprint | Simple concatenation when using different data types |
strings.Join | Concatenation of slice of string data types. |
Golang string concatenation using + operator
A simple way to join two strings is by using the +
operator. You can also use the +=
operator to do simple string joins.
This way is not the most performant solution but this will get the job done if the concatenation is for small strings which are not inside the loops
package main
import "fmt"
func main() {
str1 := "Strings are "
str2 := "Immutable in Go"
fmt.Println(str1 + str2)
// OUTPUT: Strings are Immutable in Go
str3 := str1 + "(updated) "
str3 += str2
fmt.Println(str3)
// OUTPUT: Strings are (updated) Immutable in Go
}
Output
Strings are Immutable in Go
Strings are (updated) Immutable in Go
Golang string concatenation using strings.Builder
If you want to do the string join inside the loop / do the join for a large string, you can use the more performant string.Builder methods to join multiple strings.
This was introduced from Go 1.10+ and now is the recommended way to perform optimized string manipulations.
package main
import (
"fmt"
"strings"
)
func main() {
var strBuilder strings.Builder
for i := 0; i < 10; i++ {
strBuilder.WriteString("Testing String Buffer\n")
}
fmt.Println(strBuilder.String())
}
There are multiple methods that will let you add bytes or runes to the string builder. Check out the following page for more details on Golang string builder.
Output
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Golang string concatenation using bytes.Bufferbytes.Buffer
Similar to the previous methods of joining strings, this will also let you do the join using bytes. This method is also a performant option when compared to the simple +
operator. This method is preferred when you are reading a file and want to write the output to a string.
package main
import (
"bytes"
"fmt"
)
func main() {
var strBytes bytes.Buffer
for i := 0; i < 10; i++ {
strBytes.WriteString("Testing String Buffer\n")
}
fmt.Println(strBytes.String())
}
Similar to the previous string builder method, bytes.Buffer also has some additional methods which can be useful. For more details on bytes.Buffer, check out this page - Golang bytes buffer
Output
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Testing String Buffer
Golang string concatenation using fmt.Sprint()
If you want to do the string join while printing to the console or writing to another string, this method using the fmt
package will be useful. Also you can combine different data types easily using the fmt.Sprintf method
package main
import "fmt"
func main() {
str1 := "Strings are "
str2 := "Immutable in Go"
str3 := fmt.Sprint(str1, "(updated) ", str2)
fmt.Println(str3)
// OUTPUT: Strings are (updated) Immutable in Go
numValue := 342
str4 := fmt.Sprintf("%s(updated) %s %d", str1, str2, numValue)
fmt.Println(str4)
// OUTPUT: Strings are (updated) Immutable in Go 342
}
Output
Strings are (updated) Immutable in Go
Strings are (updated) Immutable in Go 342
Golang string concatenation using strings.Join()
When you have a string slice and want to concatenate them, you can use this method.
package main
import (
"fmt"
"strings"
)
func main() {
strArray := []string{"Strings are", "Immutable in Go"}
strJoined := strings.Join(strArray, " ")
fmt.Println(strJoined)
}
Output
Strings are Immutable in Go