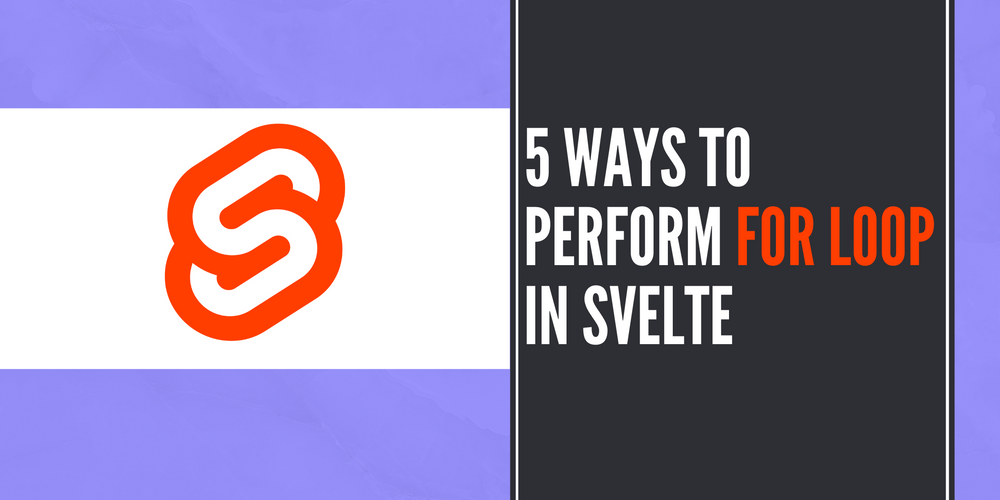
5 ways to perform for loop in Svelte (Each block)
Svelte for loop are essential things to learn. Learn about the different ways you can use each block in svelte to make things easier in your development of svelte apps-
Sriram Thiagarajan
- February 6, 2022
5 ways to perform for loop in Svelte (Each block)
Svelte provides an easy way to loop through the variable and display the data. There are multiple ways to use each block to your advantage and build awesome applications. Let’s look at some of the ways.
Each block with a simple object
We will start with the most basic usage. Consider having an array of objects and you want to display them in a list. You can start with this method.
<script>
let names = ['Name 1', 'Name 2', 'Name 3'];
</script>
<ul>
{#each names as name, index}
<li>{index} - {name}</li>
{/each}
</ul>
Each block will now use the names
variable to loop through and the name
is an alias for each item in the array. You can pass the second argument after the comma which will provide the index of the current iteration in the loop. Here we are passing the index
variable.
Each block with the keyed object
Each block allows adding a key for each of the rows to uniquely identify that particular row. This is really helpful when you are updating a particular item in the array. It is recommended to provide this key.
<script>
let names = [{id: 1, name: 'Name 1'},{id: 2, name: 'Name 2'},{id: 3, name: 'Name 3'}];
</script>
<ul>
{#each names as item, index (item.id)}
<li>{item.id} - {item.name}</li>
{/each}
</ul>
You can use any property which will uniquely identify that particular row. Here we are using the id
property in the array to identify the row.
Each block with object destructing
If you just want a few properties from the row item, you can make use of object destructing syntax. It allows you to pick a few properties from the overall item.
<script>
let names = [{id: 1, name: 'Name 1'},{id: 2, name: 'Name 2'},{id: 3, name: 'Name 3'}];
</script>
<ul>
{#each names as {id, name}, index (id)}
<li>{id} - {name}</li>
{/each}
</ul>
We are just picking the id
and name
from the item. You can then use those variables inside each block.
Each block with key-value objects
<script>
let name1 = {
name: 'Name 1',
age: 25,
gender: 'Male',
occupation: 'Software Developer'
}
</script>
<ul>
{#each Object.entries(name1) as [key, value], index (key)}
<li>{key} - {value}</li>
{/each}
</ul>
You can iterate through all the fields in the object and display them in the list. This will be useful when you are not sure of the structure of the object returned. This is possible using the Object.entries
method which will give a list of key-value pairs which we can iterate.
Each block with x number of times
If you just want to display a component a few times, you can use this method.
<ul>
{#each Array(5) as _, index (index)}
<li>Comment</li>
{/each}
</ul>
We are using the Array(length)
syntax to create a new array of 5 items that will be undefined
. Since we do not care about the value inside the array, we use _
which is a convention meaning that value is not important for our app.
Conclusion
Svelte provides a nice syntax that helps increase the productivity of the developers and keep things inside in the application. If you have any feedback or any question, feel free to join our discord
Discord - https://discord.gg/AUjrcK6eep