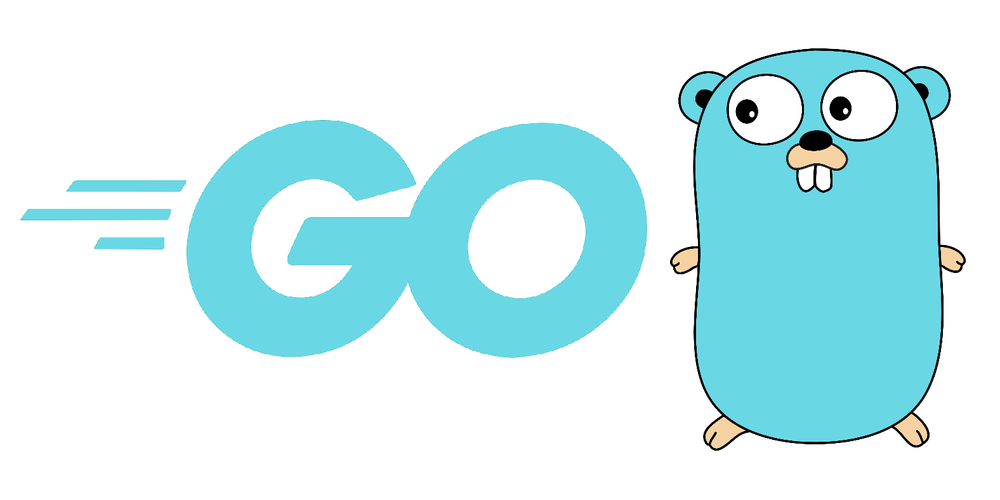
Adding and Removing dependency in Go modules
Adding and Removing dependency in Go modules with examples-
Sriram Thiagarajan
- July 23, 2021
Adding and Removing dependency in Go modules
Adding dependency is made easy with go get
command. Specify the package needed and Go will add it to the module as a dependency. Before we get to that part let’s look at how to initialize module in Go.
Topics Covered
- Initialize module
- Adding dependency
- Removing dependency
Initialize module
We can initialize the module first to create go.mod
file. This will contain details about our module
- Name of the module
- Version of Go used in the module
- Dependencies of the module
After creating a new folder for your module, run the go mod init {modulename}
command to initialize your module
go mod init helloworld
The above command creates go.mod
file with the module name “helloworld”
Adding dependency
go get
command is the standard way of adding dependencies to your modules.
Download and install particular package using the go get {package_name}
go get github.com/gofiber/fiber/v2
The above command will install the fiber package (This is just an example and don’t worry if you are not familiar with fiber)
After adding the dependency, just import it into your go file and you can start using the installed package
package main
import "github.com/gofiber/fiber/v2"
func main() {
// Staring a server
app := fiber.New()
app.Get("/", func(c *fiber.Ctx) error {
return c.SendString("Hello from fiber")
})
app.Listen(":3000")
}
For more info about go get command and its arguments, check this link
Removing dependency
When you want to remove any dependency, you need to perform a couple of steps
- Remove all the imports and code related to the package
- Run
go mod tidy -v
command to remove any unused package
From the above example, we need to remove all the places where we are using fiber.
package main
func main() {
// Staring a server
}
We just have an empty main function in our example but you get the point :)
go mod tidy -v
Output:
unused github.com/gofiber/fiber
The above command is used to remove any unused dependencies from the module. Since we have removed any reference to the installed package, it will assume that that package is not needed anymore and remove it from the dependency.
go.mod
will also be updated to remove the dependency.
Summary
- Initialize a module using
go mod init {packagename}
- Add dependency using
go get {packagename}
- Remove dependency by deleting the import references and running
go mod tidy -v
command
More details on the main and init functions in Go
Stay tuned by subscribing to our mailing list and joining our Discord community