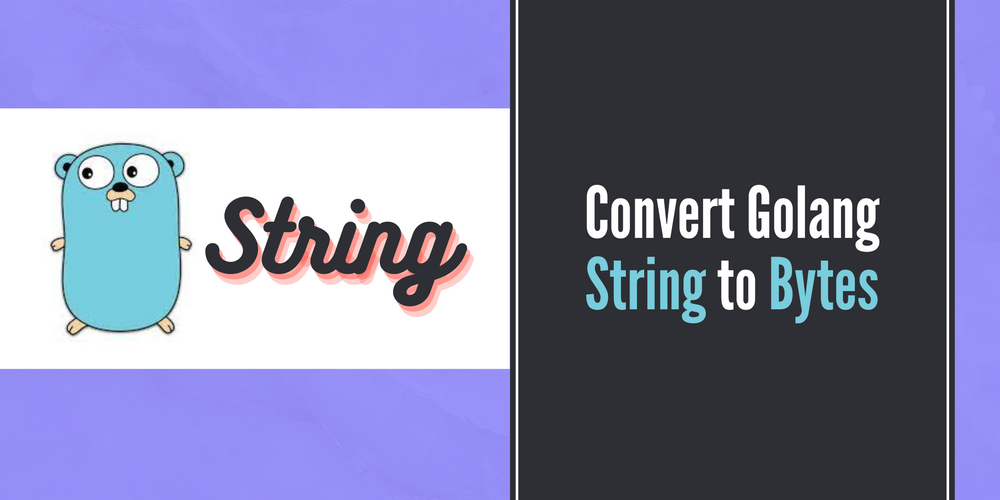
Convert string to bytes in golang
Converting string to bytes can be done using the []bytes() method-
Sriram Thiagarajan
- February 11, 2022
Convert string to bytes in golang
Strings in golang are immutables slices of bytes that are encoded in UTF-8 format. When can convert the string to bytes slice, the underlying data is the same but it will be represented in the bytes slices which can be modified unlike the string
Learn more about string and bytes in this official blog post - https://go.dev/blog/strings
How to convert string to bytes slice in golang
You can use the []byte()
function to convert the string to bytes slice
package main
import "fmt"
func main() {
strToConvert := "String data"
bytesSlice := []byte(strToConvert)
fmt.Println(bytesSlice)
}
Output:
[83 116 114 105 110 103 32 100 97 116 97]
How to convert bytes slice to string in golang
You can convert the bytes slice to string by passing the bytes slice to string
method
package main
import "fmt"
func main() {
bytesSlice := []byte{'h', 'e', 'l', 'l', 'o'}
str := string(bytesSlice)
fmt.Println(str)
}
Output:
hello