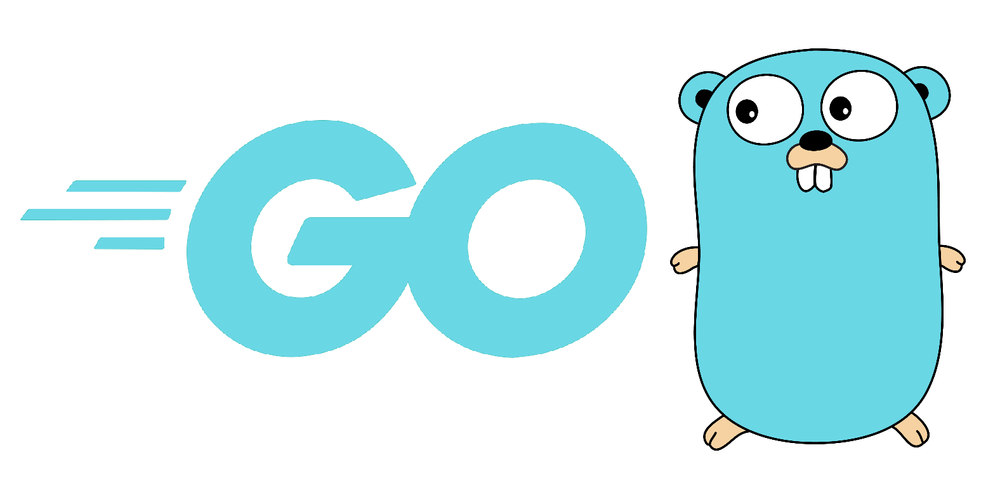
Go Switch - 6 ways of using Switch in Go
Application Development will have business logic based on a few conditions and the switch statement help provides clarity. Learn about the different ways you can use the switch statement in this article-
Sriram Thiagarajan
- August 17, 2021
6 ways of using Switch statement in Go
Basic Switch
Switch case is commonly used there are multiple condition checks to be done for a particular variable. It is easier to understand than having multiple if statements in your code.
Switch statement evaluates the expression and executes the block of code that matches from the list of possible choices
package main
import "fmt"
func main() {
status := 1
switch status {
case 0:
fmt.Println("Status - Todo")
case 1:
fmt.Println("Status - In progress")
case 2:
fmt.Println("Status - Done")
}
}
In the above program, we have a status variable that can correspond to different values based on its integer value. Business logic suggests the following
0 - Todo
1 - In progress
2 - Done
Using the switch statement, we can check the value of the status
and print the corresponding value.
Switch with default statement
There are instances when the expression being evaluated will contain a value not present in the list of cases defined. default
statement comes to our rescue by executing that case if there is no match with the other case defined.
package main
import "fmt"
func main() {
status := 1
switch status {
case 0:
fmt.Println("Status - Todo")
case 1:
fmt.Println("Status - In progress")
case 2:
fmt.Println("Status - Done")
default:
fmt.Println("Not valid status")
}
}
Switch without condition
Switch statement supports expression less statement when defining. If you just want multiple condition to be check and give those condition in the case, you can remove the expression in the switch statement
package main
import "fmt"
func main() {
status := 1
switch {
case status == 0:
fmt.Println("Status - Todo")
case status == 1:
fmt.Println("Status - In progress")
case status == 2:
fmt.Println("Status - Done")
default:
fmt.Println("Status - Not valid")
}
}
Switch with case list
Another common use case will be to have multiple expressions in a case. Multiple expressions are separated by commas.
package main
import "fmt"
func main() {
status := 4
switch status{
case 0:
fmt.Println("Status - Todo")
case 1:
fmt.Println("Status - In progress")
case 2, 3, 4:
fmt.Println("Status - Done")
default:
fmt.Println("Status - Not valid")
}
}
Switch with fallthrough statement
Execution of the statements in switch goes from top to bottom. When a particular case evaluates to be true, execution of the statement takes place. Control jumps out of the switch after execution of the successful case. fallthrough
statement is used to transfer the execution of the code to the first statement of the case after the executed case
Fallthrough can be used when you want to execute the successive case irrespective of its condition
package main
import "fmt"
func main() {
switch status := 3; {
case status < 2:
fmt.Println("Status is not done")
case status < 4:
fmt.Println("Status is done")
fallthrough
case status > 6:
fmt.Println("Status is done but has some issues")
}
}
Output
Status is done Status is done but has some issues
Things to note about fallthrough
- Fallthrough execute the next case even if the case will evaluate to false. Above example shows that the last case is executed but the condition
status > 6
is false. - Fallthrough has to be the last statement in your case
- Fallthrough cannot be present in the last case
Switch with break statement
Execution of the case statements can be stopped by using the break
statement.
package main
import "fmt"
func main() {
switch status := 2; {
case status < 2:
fmt.Println("Status is not done")
case status < 4:
fmt.Println("Status is done")
if (status == 2) {
break;
}
fallthrough
case status > 6:
fmt.Println("Status is done but has some issues")
}
}
Output
Status is done
In the above program, when the status is 2
, then the second case gets executed. if
condition becomes true and so break
is called which sends the control to the end of the switch statement without running the fallthrough
statement
Similarly, you can break from a labeled loop by specifying the label after the break statement
package main
import (
"fmt"
"math/rand"
)
func main() {
outerloop:
for {
status := rand.Intn(6)
switch status {
case 0,1,2:
fmt.Println("Waiting for status to complete")
case 3,4,5:
fmt.Println("Status is done")
break outerloop
}
}
}
for
loop without a condition will execute infinite loop until it runs into a break statement. For more info you can have a loop into this blog post.
https://www.eternaldev.com/blog/go-learning-for-loop-statement-in-depth/
status
variable will contain a random value between 0 to 5 for each loop iteration. If that value is less than 3, “Waiting for status to complete” is printed. If the value is greater than or equal to 3, “Status is done” is printed and we break the outer for loop.
So this program executes until we get values from 3 to 5 from the random value function.
Practical Assignment - Try these out
-
Simple app which takes the name of the task and status of the task from the user, and outputs the remaining status for the task using the Switch statement
- Status list - Created, Sprint Ready, Work in Progress, Completed, Deployed, Verified
- User input - “Feature A”, “Work in Progress” - Output - 3 steps are pending
- User input - “Feature C”, “Created” - Output - 5 steps are pending
Use the live code editor below to work on the assignment
Summary
Stay tuned by subscribing to our mailing list and joining our Discord community