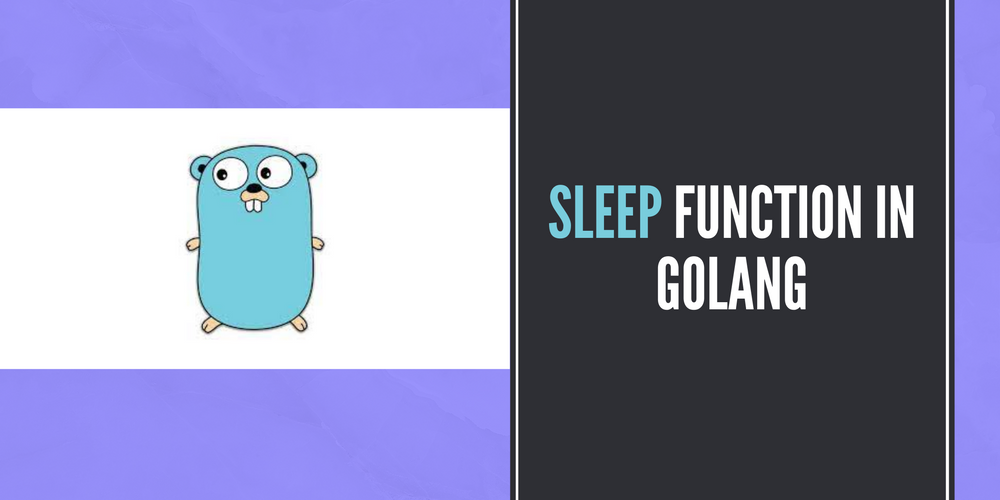
How to pause execution using the sleep function in Golang
Learn about the Sleep function from the time package to pause the execution of the thread. Also learn to make the interesting typewriter effect in the console using the sleep method-
Sriram Thiagarajan
- February 18, 2022
How to pause execution using the sleep function in Golang
The sleep function from the time package is used to pause the execution of the current thread for a particular duration of time. This can be helpful when you are performing some asynchronous activity and want to wait for a few seconds before executing some code.
What does the sleep function do?
Sleep()
function is used to pause the current go-routine for the specified amount of time. After that, it will continue the execution of the code which is provided. It has to be imported from the time
package. It has only one argument where you have to pass the duration. You can do this using the primitives like time.Second
or time.Minute
from the time package
import "time"
func main() {
time.Sleep(30 * time.Second) // Sleeps for 30 seconds
}
Example of sleep function
Simple function to illustrate the point of the thread being paused when using the sleep method.
package main
import "fmt"
import "time"
func main() {
x := 25
y := 600
fmt.Println("Processing the numbers....")
time.Sleep(5 * time.Second)
fmt.Printf("Sum of x + y = %d", x + y)
}
Why use the sleep function
Sleep function is used when multiple threads are running and you want to wait for some time in this thread to let other threads finish. It is an important tool when you are working with asynchronous programming during debugging as well. It can be used to create timers and other utilities as well.
Creating a Typewriter effect with sleep function
package main
import "fmt"
import "time"
func main() {
typewriter("This text will print in a typewriter effect")
}
func typewriter(s string) {
currentIndex := 0
for ;currentIndex < len(s); currentIndex++ {
fmt.Printf("%c", s[currentIndex])
time.Sleep(100 * time.Millisecond)
}
}
Each letter of the output will be printed after a 100
millisecond delay. This will create an illusion of the text being printed in a typewriter effect. This can be used to add a more dramatic effect if you are telling a story to the user.
Join our discord for more questions and discussions
Discord - https://discord.gg/AUjrcK6eep