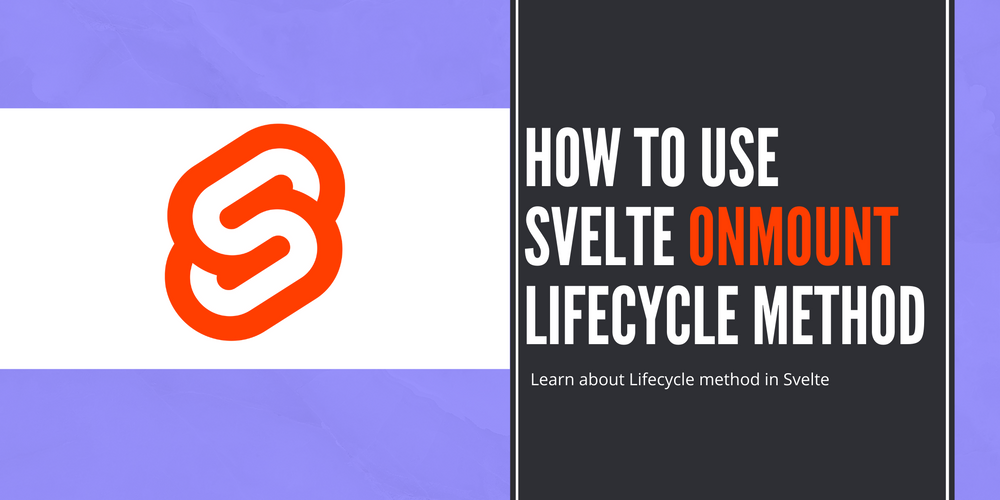
How to use Svelte onMount Lifecycle method
onMount provides a way to run a function when the component is loaded to the DOM. This can be used to initialize values, calling API to load some data, perform actions which needs to run only once.-
Sriram Thiagarajan
- December 31, 2021
How to use Svelte onMount Lifecycle method
Introduction
As a frontend developer, we need to know when the component is loaded and removed from the browser DOM to execute certain logic. This is very easy to do when you understand the lifecycle events of the framework. Svelte offers a handful of events to do these changes.
What is onMount Svelte?
onMount provides a way to run a function when the component is loaded to the DOM. This can be used to initialize values, calling API to load some data, perform actions which needs to run only once.
Template of onMount
<script>
import {onMount} from "svelte";
onMount(() => {
// Write you code here....
// This is executed when the component is loaded into the DOM
// Optional return function
return () => {
// This is executed when the component is removed from the DOM
}
})
</script>
Examples for onMount
Let’s look at some of the examples
- Fetching data from an API
- Processing data
- Accessing DOM Elements
- Adding callback to event
Fetching data from an API
<script>
import {onMount} from 'svelte';
let posts = [];
onMount(async () => {
let data = await fetch('https://jsonplaceholder.typicode.com/posts?_limit=10');
let postData = await data.json();
posts = [...postData];
});
</script>
{#each posts as post}
<h3>{post.title}</h3>
{/each}
We are creating a variable called posts
to hold the data returned from the API. We can then use the onMount
to call the API. Adding the async
keyword before the onMount callback allows users to use the await
syntax. We are using the fetch
method to call the endpoint which will return the list of posts.
As we are using the await
keyword, the execution of the next line is done only after the endpoint result is obtained. After that, we can convert the data into json
format and we are appending to the posts
variable.
Processing data
<script>
import {onMount} from 'svelte';
let names = ['first', 'second', 'third'];
onMount(() => {
names = names.map((item) => {
return item.toUpperCase();
})
});
</script>
{#each names as name}
<h3>{name}</h3>
{/each}
We are using simple logic to transform the data in the onMount in this example. we are converting the string to uppercase on the component load.
Accessing DOM Elements
<script>
import {onMount} from "svelte";
let emailInput;
let email = "";
onMount(() => {
emailInput.focus();
})
</script>
<input type="email" name="email" bind:value={email} bind:this={emailInput}/>
When we are creating a new form page and you want the user to start entering the text directly, you can bind the input element to a variable using bind:this
syntax. This will give access to the variable to call any methods on that element. We can safely call the method on the onMount
since we know that the element has been rendered on the screen when the onMount
is executed.
Adding callback to event
<script>
import {onMount} from "svelte";
let count = 0;
const keydownListener = (event) => {
if (event.keyCode == 32) {
count = count + 1;
}
}
const resetCount = () => {
count = 0;
}
onMount(() => {
window.addEventListener('keydown', keydownListener)
return () => window.removeEventListener('keydown', keydownListener);
})
</script>
<h2>
"Space" key has been pressed {count} times
</h2>
<button on:click={resetCount}>Reset</button>
We can add a callback to the window event called keydown
to listen to any keystroke which the user is performing. We can then check if the keyCode
value is 32, then increment the counter value. onMount
can return another function which is executed when the component is removed from the DOM. We are removing the event listener to avoid any memory leak after the component is removed from the DOM
Things to note about onMount
- onMount function can be called from a external module (ie. External module which has
onMount
function defined will be executed when you are calling that function inside your component.) - onMount function does not execute in Server Side Rendering (SSR)
Conclusion
Lifecycle methods are really helpful and svelte makes it easy to add these to the component.