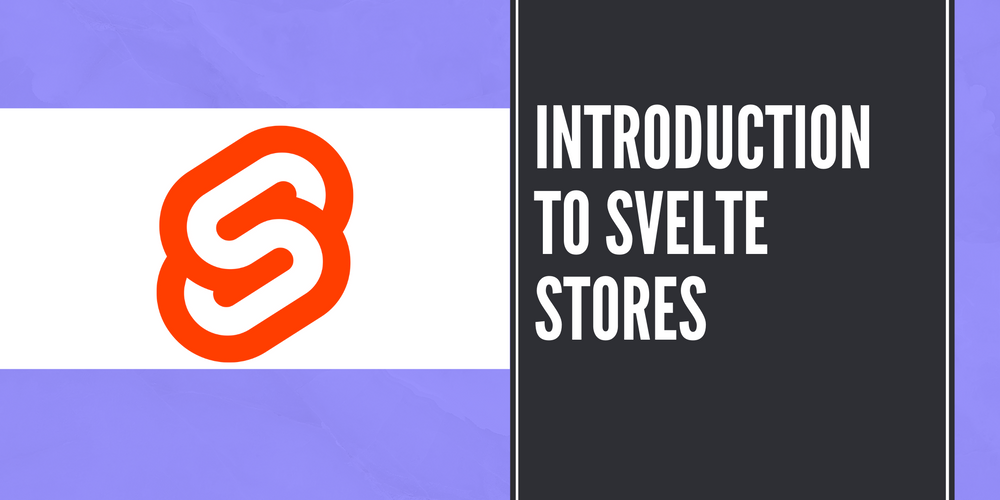
Introduction to Svelte Readable Store
Store in Svelte are a very good way to keep the data and component logic separate and have access to those data across multiple components. In this article, we will learn how to make use of readable store from Svelte-
Sriram Thiagarajan
- December 24, 2021
Introduction to Svelte Readable Store
Store in Svelte are a very good way to keep the data and component logic separate and have access to those data across multiple components. In this article, we will learn how to make use of readable store from Svelte
When to use Svelte Readable store ?
You might have some data which needs to be shared across component but components should not update the values. You can use this when building a stock market app where the data refreshes every second. The components should not update the data but can read the data to display in the UI.
To understand this, Let’s build an app which will get a new user data every 10 seconds and displays the user data on the screen.
Creating a readable store
Let’s start by creating an new Svelte with Typescript project using Vite. You can follow this post to create a new project
https://www.eternaldev.com/blog/build-and-deploy-apps-with-svelte-and-vite/
After setting a new project, you can add a new folder called stores
and create a new file called userStore.ts
import { readable } from "svelte/store";
type UserData = {
id: string;
firstname: string;
lastname: string;
}
export const user = readable(initialData, (set) => {
});
Let’s break down the code
- We are importing
readable
from the “svelte/store” - We are adding
UserData
typescript type which will be used to store the data for the user.
Calling API inside readable store
Since its readable store, we can only update the value of the store inside the store function. In our case, let’s call an API to fetch the data every 5 seconds and update the readable store.
readable
store takes an initial value as the first argument. Second argument is the start
function which has a set
callback function. set
callback is used to set the value of the store and cannot be used outside since its read-only for other components.
start
function return a stop
function. This is the interesting part as you can use these to perform cleanup when you store variable is not used by anyone.
The
start
function is called when the store gets its first subscriber andstop
is called when the last subscriber unsubscribes.
const initialData: UserData = {id: '123', firstname: 'First Name', lastname: 'Last Name'};
export const user = readable(initialData, (set) => {
const userApiInterval = setInterval(() => {
fetch('https://randomuser.me/api/').then((data) => data.json()).then((user) => {
const {first, last } = user.results[0].name;
const {value} = user.results[0].id;
const newUser: UserData = {
id: value,
firstname: first,
lastname: last
}
set(newUser);
}).catch((ex) => {
set(null);
})
}, 5000);
return () => clearInterval(userApiInterval)
})
In our example, we are calling the setInterval
in the start function and calling an API which will return random user data. We are using the set
function to set the new data.
The start
function returns a stop
function which call the clearInterval
in this case so as to cleanup the interval in the browser when there is no subscribers to this store.
If you want to know about the API used here to get random user data, check this link. It has a lot more data about the user than what is used in this example.
Create component to use readable store
Create a new UserDisplay.svelte
file in the lib folder.
<script lang="ts">
import {user} from '../store/userStore';
</script>
{#if $user != null}
<h1>{$user.firstname} {$user.lastname}</h1>
{:else}
<h1>Error while loading the data</h1>
{/if}
- Just import the store from
../store/userStore
and the use the variable - Check if the user value is not null and display the value
- If the value is null, show error message
Result
Conclusion
Readable store is a useful tool in the toolbox for svelte developers. I can help in reducing the code size and help prevent unwanted updates to the store data.