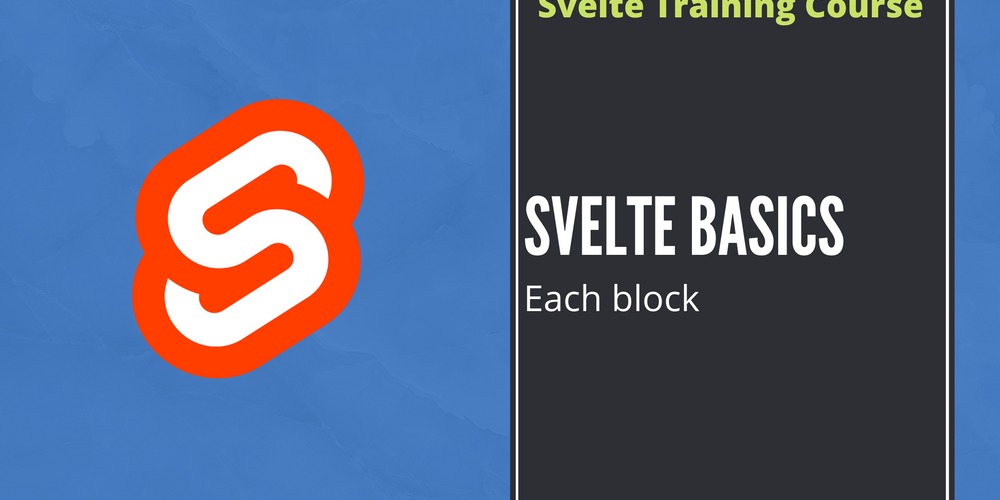
Svelte Basics - Each block - Part 5
Svelte Each block can be used to loop through the items in an array to perform some task. Usually, it is used to render a list of items/ display the same element multiple times.-
Sriram Thiagarajan
- April 3, 2022
Svelte Basics - Each block - Part 5
This post is a continuation of the course on Svelte. we would recommend going through the rest of the posts in the order to get the maximum out of this course
Previous post - Svelte - If/ Else/ Else if Blocks
Why do we need Each block in Svelte
Loops are an essential part of programming which let’s use to automate a simple task many times. Svelte Each
block can be used to loop through the items in an array to perform some task. Usually, it is used to render a list of items/ display the same element multiple times.
How to define Each block in Svelte
Each block syntax is pretty simple and can be used to perform outstanding results. You can use the following syntax
{#each [array] as [array_item], [index]}
// Looping code here
{/each}
#each
represents the start of the loop[array]
represents the array item to loop over. This will contain all the items which we need to iterate.[array_item]
represents the single item in the array. This item will be automatically changed to the current item in the iteration. When the 1st iteration of the loop is taking place, this will contain the first item and when the 2nd iteration happens it will contain the 2nd item in the array.[index]
represent the current iteration index. Similar to thearray_item
, the value will keep modified when there is a change in the iteration of the loop
Building a Navbar using Each block
Let’s put the learning in action and build a nav bar using the Each block. We will have to define the items of the nav bar at the start and then loop through them using the Each block.
<script>
let menu_items = [{ name: 'Home', url: '/'}, {name: 'Contact', url: '/contact'}];
</script>
<nav>
{#each menu_items as menu_item}
<a href={menu_item.url}>{menu_item.name}</a>
{/each}
</nav>
<style>
nav {
display: flex;
justify-content: center;
gap: 20px;
}
</style>
Svelte Nav Bar - Each block REPL
Getting the index of items in Each block
Getting the index of each item will be helpful if you want to perform some modification to the array content inside the loop. Consider the example of adding a delete button inside the loop to remove an item.
<script>
let things = ["Buy Grocery", "Make Food", "Cleaning"];
const removeItem = (index) => {
if (index < things.length) {
things = [...things.slice(0, index), ...things.slice(index + 1)]
}
}
</script>
<ul>
{#each things as thing, index}
<li>
{index} - {thing}
<button on:click={() => removeItem(index)}>Done</button>
</li>
{/each}
</ul>
Svelte Each block with Index - REPL
Conclusion
To learn more about different ways to use Each block in Svelte, Check out 5 ways to perform Each block