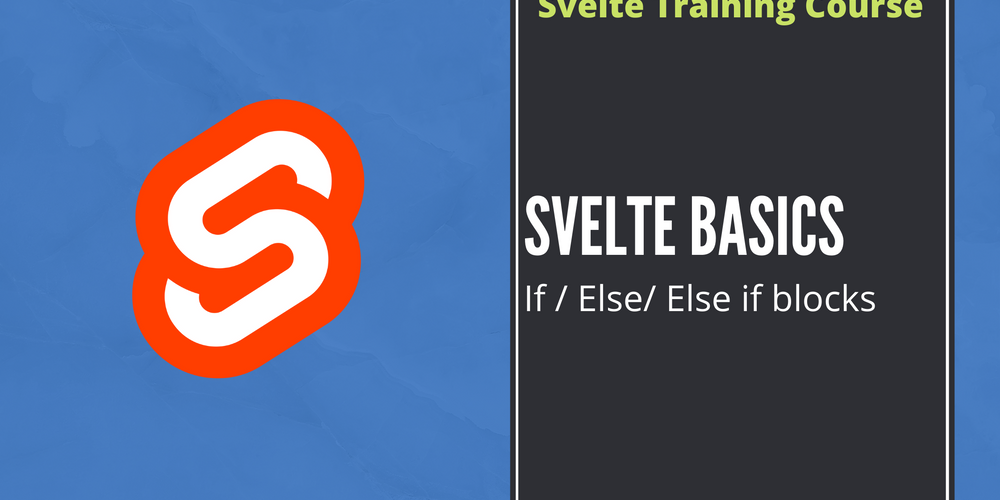
Svelte Basics - If / Else / If Else block - Part 4
Learn about how to add if and else block syntax in Svelte-
Sriram Thiagarajan
- March 25, 2022
Svelte Basics - If / Else / If Else block - Part 4
This post is a continuation of the course on Svelte. If you haven’t read the previous parts of the series, we would recommend going through the post in the order.
Previous post - Svelte Reactivity
Why do we need If block in Svelte?
Svelte if
block is useful to display Svelte/HTML elements based on some condition. Rendering some part of the HTML based on some condition is also known as Conditional Rendering.
How to define an If block in Svelte
If
block can be simple with just one condition or you can have multiple conditions using the else
and else if
block. These help in making the conditional rendering in Svelte really simple.
{#if [condition]}
// HTML goes here
{:else if [condition]}
// HTML goes here
{:else}
// HTML goes here
{/if}
- It starts with one or more conditions that can be added to the first line.
- You can have one or more lines of simple HTML / Svelte components after the
if
condition else if
condition helps in adding more logic if the firstif
block is falseelse
condition executes when all the other conditions have been executed to false.
Svelte If block
Svelte offers an easy way to hide and show HTML elements based on some conditions. Let’s see an example of how to add an if
block
<script>
let showComments = true;
</script>
{#if showComments}
<h3>Comments blocks</h3>
{/if}
Svelte Else block
If you have an else condition and want to show different HTML elements, you can use the else syntax in Svelte.
<script>
let showComments = false;
</script>
{#if showComments}
<h3>Comments blocks</h3>
{:else}
<h3>Something else</h3>
{/if}
Svelte Else-If block
When having multiple conditions, you can use the else if syntax to cover all the different scenarios.
<script>
let shape = 'circle';
</script>
{#if shape == 'square'}
<h3>Sqaure</h3>
{:else if shape == 'circle'}
<h3>Circle</h3>
{:else if shape == 'triangle'}
<h3>Triangle</h3>
{:else}
<h3>Unknown Shape</h3>
{/if}
Conclusion
If / Else syntax makes it easy to write logic inside Svelte components as these are commonly used when it comes to real-world applications